COM413: Mobile Application Development Practical Workbook 1 Guidance
Category | Assignment | Subject | Programming |
---|---|---|---|
University | Ulster University | Module Title | COM413: Mobile Application Development |
Question 1. Variables and Data Types
Create a new class and hard code the following five variables:
Name (use an initial and surname), Age, Salary and“isEmployed”.
Each variable must use a differentDataTypeto store each variable, print the following statement using variables:
“J Smithis 25 years old, is currently employedand has a salary of£21,000 per year.”
Modify the code to accept user input for each variable. These are indicated as bold.
Use the scanner class: Scanner scanner= new Scanner(System.in);
Question 2. Basic Arithmetic
Create a new class and hard code the following:
int a, int b, sum, difference, product and quotient.
Sum = a + b, difference = a – b, product = a x b and quotient is a/b.
Using the values: a = 10 and b = 5
Print the results as:
Sum: 15
Difference: 5
Product: 50
Quotient: 2.0
Modify the code to accept user input for each value and allow the user to specify their choice of arithmetic.
Question 3. Conditional Statements
Create a new class which will check if a value is odd or even and will print the result.
Use the modulus operator (num % 2 == 0) to check if the value is odd or even.
You can hard code the num value to check if it works and then upgrade your code to use the Scanner class so the user can input a number.
Modify the code to ask the user if they want to continue entering numbers using Y/N and based on the answer allow the user to enter more numbers.
Question 4. Loops
Create a new class which will Print numbers from 1 to 10 using a while loop.
This is called a conditional loop.
Now, print numbers from 1 to 10 using a for loop.
This is called an unconditional loop.
Conditional looping: Computation continues indefinitely until the logical condition is true. Unconditional looping: The number of repetition known in advance or repetition is infinite.
Now, iterate through this array using a for-each loop:
String[] fruits = {“Apple”, “Banana”, “Orange”, “Grapes”};
Modify this code so that you populate a list of movies, from the user using a loop and then print these films in a list using a for-each loop.
Question 5. Methods
Create a new class, within this class you will have a method which will take two numbers as parameters and return the sum of these values.
Again, you can hard code to test, but use the Scanner class to ask the user for two values. Print the result as such:
Enter a value for A:
5
Enter a value for B:
8
Sum: 13
Modify the code and add a subtract method, product method and quotient method.
Question 6. String Manipulation
Create a new class, use the scanner to ask the user for their name. Once the user inputs their name perform the following functions to retrieve the following information:
Enter your name: Person
Hello, Person
Length: 6
Uppercase: PERSON
Lowercase: person
Reverse: nosrep
Contains ‘@’: false
Note: if you store the name as a String Variable, it becomes an object, you can perform a number of actions on the string by calling the appropriate method, simply type the name of the variable e.g. name and then enter a full stop, this will give you a list of methods. Methods are performed on objects.
Question 7. Objects – 10 marks
Create a new class, and enter the following code:
public class Practical7 { public static void main(String[] args) { }} class Person { String name; int age; void introduce() { System.out.println(“Hello, my name is ” + name + ” and I am ” + age + ” years old.”); }}
Within this project, a new class is introduced. This class allows objects to be created. Objects allow us to use the same code to create similar objects but with different types. Using people as an example, we create a class Person, we use two variables name and age to store each individuals information. We then use a constructor to create a new instance of a class and use the methods contained within that class to simplify the creating a new person.
Complete the code so the following information will be shown:
Hello, my name is Alice and I am 30 years old.
Hello, my name is Bob and I am 25 years old.
Next use the scanner class and create a new person:
Hello, my name is Alice and I am 30 years old.
Hello, my name is Bob and I am 25 years old.
Enter name:
George
Enter age:
23
Hello, my name is Person and I am 53 years old.
Question 8. Basic Calculator
Create a new class. This practical requires you to create a basic calculator, which should look something like this:
Calculator Application
1. Add
2. Subtract
3. Multiply
4. Divide
5. Exit
Enter your choice:
The application should use a while loop and a switch case statement. While the user doesn’t enter the number five, the application will run again, to exit the program the user will enter the number five. There should be code to stop the user dividing by zero and inputting a number that isn’t an option.
Question 9. Shapes Calculator
Create a new class. This practical requires you to create a shapes calculator that prompts the user to input a shape (square, rectangle, triangle, or circle) and calculates the area and perimeter for each shape using separate methods. Here are the formulas used to calculate the area, and in the case of the square and rectangle, the perimeter.
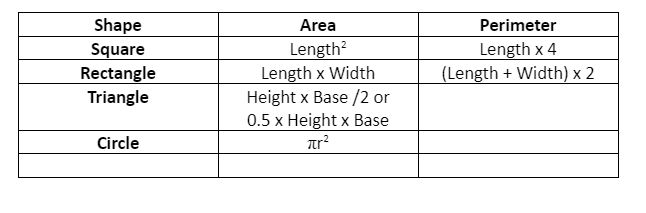
Question 10. Binary to Decimal converter
Create a program which prompts the user to input a binary number and then converts it to its decimal equivalent using the binary-to-decimal conversion method.
a. Binary values can be read as an array of 1s and 0s. 1 in the array is represented as the value in the top line. Write a program which will take a 4-bit code and calculate the decimal equivalent. The numbering system is below:

For example: a binary code of 1111 would be equal to 8+4+2+1 = 15.
b. Scale this up to 8 bits.

For example: 00110011 would equal 32+16+2+1 = 51.
The binary here isn’t important, you are looking through the array and using the 1s and 0s to give you a corresponding value